java-备忘录模式
本文最后更新于:3 个月前
备忘录模式
基本介绍:
- 备忘录模式(Memento patten)在不破坏封装性的前提下,捕获一个对象的内部状态,并在该对象之外保存这个状态。这样以后就可以将该对象恢复到原先保存的状态。
- 现实生活中的备忘录是用来记录某些要去做的事情,或者是记录已经达成的共同意见的事情,以防忘记了。而在软件层面,备忘录模式有着相同的含义,备忘录主要用来记录一个对象的某种状态,或者某些数据,当要做回退时,可以从备忘录对象里获取原来的数据进行恢复操作。
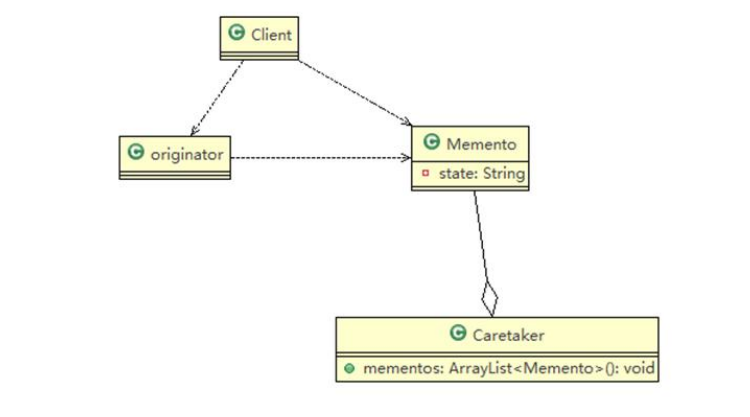
代码实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class Memento { private String state;
public Memento(String state) { super(); this.state = state; }
public String getState() { return state; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class Originator {
private String state;
public String getState() { return state; }
public void setState(String state) { this.state = state; } public Memento saveStateMemento() { return new Memento(state); } public void getStateFromMemento(Memento memento) { state = memento.getState(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class Caretaker { private List<Memento> mementoList = new ArrayList<>(); public void add(Memento memento) { mementoList.add(memento); } public Memento get(int index) { return mementoList.get(index); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public class Client {
public static void main(String[] args) {
Originator originator = new Originator(); Caretaker caretaker = new Caretaker(); originator.setState(" 状态#1 攻击力 100 "); caretaker.add(originator.saveStateMemento()); originator.setState(" 状态#2 攻击力 80 "); caretaker.add(originator.saveStateMemento()); originator.setState(" 状态#3 攻击力 50 "); caretaker.add(originator.saveStateMemento()); System.out.println("当前的状态是 =" + originator.getState()); originator.getStateFromMemento(caretaker.get(0)); System.out.println("恢复到状态1 , 当前的状态是"); System.out.println("当前的状态是 =" + originator.getState()); } }
|
游戏案例代码实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class Caretaker {
private Memento memento;
public Memento getMemento() { return memento; } public void setMemento(Memento memento) { this.memento = memento; }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| public class GameRole {
private int vit; private int def; public Memento createMemento() { return new Memento(vit, def); } public void recoverGameRoleFromMemento(Memento memento) { this.vit = memento.getVit(); this.def = memento.getDef(); } public void display() { System.out.println("游戏角色当前的攻击力:" + this.vit + " 防御力: " + this.def); }
public int getVit() { return vit; }
public void setVit(int vit) { this.vit = vit; }
public int getDef() { return def; }
public void setDef(int def) { this.def = def; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class Memento {
private int vit; private int def; public Memento(int vit, int def) { super(); this.vit = vit; this.def = def; } public int getVit() { return vit; } public void setVit(int vit) { this.vit = vit; } public int getDef() { return def; } public void setDef(int def) { this.def = def; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class Client {
public static void main(String[] args) { GameRole gameRole = new GameRole(); gameRole.setVit(100); gameRole.setDef(100); System.out.println("和boss大战前的状态"); gameRole.display(); Caretaker caretaker = new Caretaker(); caretaker.setMemento(gameRole.createMemento()); System.out.println("和boss大战~~~"); gameRole.setDef(30); gameRole.setVit(30); gameRole.display(); System.out.println("大战后,使用备忘录对象恢复到站前"); gameRole.recoverGameRoleFromMemento(caretaker.getMemento()); System.out.println("恢复后的状态"); gameRole.display(); } }
|
注意事项和细节
- 给用户提供了一种可以恢复状态的机制,可以使用户能够比较方便的回到某个历史的状态。
- 实现了信息的封装,使得用户不需要关心状态的保存细节。
- 如果类的成员变量过多,势必会占用比较大的资源,而且每一次保存都会消耗一定的内存、这个需要注意。
- 使用的应用场景
- 后悔药
- 游戏存档
- Windows里的ctrl+z
- 浏览器的回退
- 数据库的事务管理
- 为了节省内存,备忘录模式可以结合原型模式一起食用
本博客目前大部分文章都是参考尚硅谷或者马士兵教育的学习资料!